10 React Hooks Every Developer Should Know in 2024
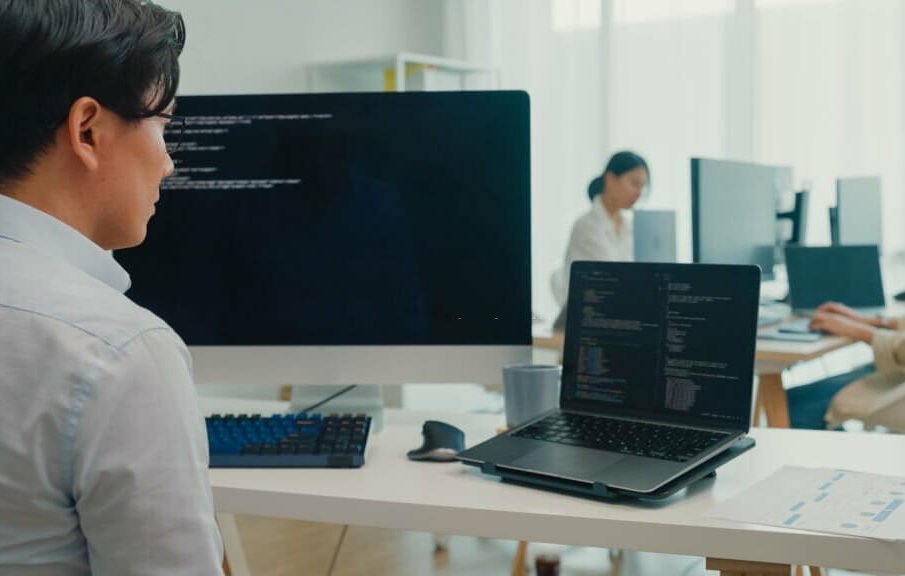
React Hooks
React Hooks are basic JavaScript functions that enable functional components to access other React features that were only earlier accessible to class components. Introduced in React 16.8, hooks brought a new revolution in how developers build components and manage states in React applications. There are a bunch of different hooks that serve different purposes in easing your React application development journey. Most of these hooks are provided by React as built-in tools such as useState, useEffect, useContext, and more.
With React Hooks, your React application gets a direct API reach to most React features that helps components eliminate the dependency on class components to achieve the desired results. Since there is a wide variety of hooks, and they all have immense potential to be leveraged, we will discuss some of the top React Hooks that you should know before you hire ReactJS developers for your next web development project –
Types of React Hooks to Know in 2024
React provides many built-in hooks that it knows will be useful for most React.js development projects. Today, we will be discussing some of the top React Hooks that can benefit any web development project –
useState
The useState hooks is the most commonly used React hook. Its purpose is to allow developers to add state to their functional components, giving them access to features previously only made available to class components, like maintaining state when a component would re-render.
When you use the useState hook, it will take an initial state value and return an array with two elements – the current state value and a function for updating it.
Here’s an example using useState:
const[count, setCount] = useState(0);
When you use the useState feature this way, it provides you a count to store your state value and a setCount function to update it. The setCount triggers a re-render whenever called and passes the new state value to the component.
This React Hook is useful whenever you must maintain and update the state for a functional component like API data, counter value, input values, and more. You won’t have to turn the functional component into a class to access the local component state.
useEffect
useEffect allows you to handle side effects in your components. Some examples of side effects are fetching API data directly and updating the DOM and timers.
useEffect accepts two arguments – a callback function and an array of dependencies. The callback will run after render and whenever one of the dependencies changes.
Here’s an example usage fetching API data:
useEffect(() => {
fetchAPIdata();
}, []);
The empty array means it will only run once on the mount. You could also pass in props or state values to re-run when those change.
This allows you to replicate componentDidMount and componentDidUpdate behavior in a functional component. useEffect hooks make it easier to handle complex side effects directly within your components.
useContext
If useContext allows you to consume context in a functional component. It provi a way to pass data down the component tree without passing props at every level.
First, you create a context object:
const AppContext = React.createContext();
Then provide this at the top level of your app using the context provider component:
<AppContext.Provider value={data}>
Finally, consume the context in any nested component using useContext:
const data = use context(AppContext);
useContext eliminates the need to pass props several levels to get data to deeply nested components. This makes it easier to access global data anywhere in your app.
seducer
useReducer allows you to manage local state with a reducer function instead of a setState call. This is useful for more complex state management.
useReducer takes in a reducer function and an initial of state. The reducer receives the current state and returns an action and the updated state:
const [state, dispatch] = useReducer(reducer, initialState);
You can then dispatch actions to your reducer to update the state:
dispatch({type: ‘INCREMENT’});
useReducer is like a mini Redux built into React. It helps reduce boilerplate for complex state transitions that require more than just updating state values.
use callback
use callback allows you to memoize callback functions so that they aren’t recreated on every render.
This hook accepts a callback function and a dependencies array. use callback will return a memoized callback that only changes if one of the dependencies updates:
const memoizedCallback = use callback(
() => {
doSomething();
},
[someProp],
);
Now memoizedCallback will not change identity unless someProp changes. This is useful for passing callbacks to optimized child components that rely on reference equality to prevent unnecessary renders.
useMemo
useMemo is similar to useCallback, but for memoizing values instead of functions. It accepts a function to run for calculating the memoized value and a dependencies array.
Here’s an example:
const memoizedValue = useMemo(() => computeValue(a, b), [a, b]);
Now memoizedValue will not recalculate until a or b change. This is useful for improving performance by avoiding expensive calculations on every render.
useRef
useRef returns a mutable ref object with a current property initialized to the passed argument. .This object that was returned will stay around as long as the component does.
A common use case is attaching it to a DOM element:
const inputRef = useRef();
<input ref={inputRef} />
You can then access the DOM element through inputRef.current.
useRef is also useful for keeping mutable values that don’t cause re-renders when updated. This can keep previous state values, mutable timers, and more.
useImperativeHandle
useImperativeHandle allows you to customize the instance value exposed to parent components when using ref.
It should be used with forwardRef:
function Child(props, ref) {
useImperativeHandle(ref, () => ({
greeting: () => console.log(‘hello’)
}))
}
export default forwardRef(Child);
The parent can then call greeting() on the child’s ref.
const childRef = useRef();
childRef.current.greeting();
useImperativeHandle allows exposing custom instance values and methods on a ref. It should be used in conjunction with forwardRef.
useLayoutEffect
useLayoutEffect is identical to useEffect, except it runs synchronously after DOM mutations. Use it for cases that need to read the layout from the DOM and synchronously re-render.
Here’s an example of usage:
useLayoutEffect(() => {
const element = document.getElementById(‘myElement’);
const left = element.getBoundingClientRect().left;
// do something with left
}, [myElement])
This will run after render and after the DOM has been updated. This allows you to synchronously measure the DOM and do something before the browser can paint.
Most effects don’t need to be synchronous. But for the rare cases that do, like measuring DOM nodes, useLayoutEffect is useful.
useDebugValue
useDebugValue allows you to display a label for custom hooks in React DevTools.
Here’s an example of usage:
function useCustomHook() {
useDebugValue(‘custom hook!’);
return something;
}
Now, in DevTools, this hook will display the debug label provided.
useDebugValue is not used often but can help provide more context in DevTools when working with complex custom hooks.
Conclusion
These are some of the most common and useful React hooks you should know as a developer. React hooks unlock great power and flexibility for functional components, eliminating the need for class components in many cases.
Hire dedicated developers with knowledge of leveraging best hooks for cleaner component logic, better organization, and improved performance. Their simple API unlocks access to React features like state, lifecycle methods, context, and refs from function components.
Hooks continue to evolve and improve in every React release. But mastering the core hooks above will provide a great foundation for building React applications the “right way.” Keep these hooks in mind, and you’ll be on your way to React mastery. Read more